문제 설명
문제 풀이
from pprint import pprint
def solution(friends, gifts):
friends = sorted(set(friends))
give_cnt = {friend : 0 for friend in friends} # 준 횟수
receive_cnt = {friend : 0 for friend in friends} # 받은 횟수
present_cnt = {friend : 0 for friend in friends} # 선물 지수
next_month_cnt = {friend : 0 for friend in friends} # 다음달에 받는 횟수
gr_records = {
friend: {other: [0, 0] for other in set(friends) - {friend}}
for friend in friends
} # 주고 받는 횟수 기록 : 딕셔너리 - 초기화
for gift in gifts:
giver, receiver = gift.split(" ")
give_cnt[giver] += 1
receive_cnt[receiver] += 1
gr_records[giver][receiver][0] += 1
gr_records[receiver][giver][1] += 1
# 주고 받는 횟수 기록
# pprint(gr_records)
give_cnt_list = list(give_cnt.values())
receive_cnt_list = list(receive_cnt.values())
present_cnt_list = list(present_cnt.values())
present_cnt_list = [give_cnt_list[i] - receive_cnt_list[i] for i in range(len(friends))]
present_cnt = {friend : present_cnt_list[i] for i, friend in enumerate(friends)}
for i in range(len(friends)-1):
friend = friends[i]
# print(friend)
others = friends[i+1:]
# print(others)
for other in others:
AtoB = gr_records[friend][other][0] # A 가 B 에게 준 것
BtoA = gr_records[friend][other][1] # A 가 B 에게 받은 것
if AtoB > BtoA:
next_month_cnt[friend] += 1
elif AtoB < BtoA:
next_month_cnt[other] += 1
elif AtoB == BtoA :
if present_cnt[friend] > present_cnt[other]:
next_month_cnt[friend] += 1
elif present_cnt[friend] < present_cnt[other]:
next_month_cnt[other] += 1
else:
next_month_cnt[other] += 0
# print(next_month_cnt)
answer = max(list(next_month_cnt.values()))
return answer
전체 풀이 코드입니다.
먼저 준 횟수, 받은 횟수, 선물 지수, 다음 달에 받는 횟수, 주고 받은 전체 레코드를
give_cnt
receive_cnt
present_cnt
next_month_cnt
gr_records
로 정의 했고
for gift in gifts:
giver, receiver = gift.split(" ")
gifts 에서 각 gift 의 giver와 receiver를 정의해주었습니다.
split(" ") 로 중간에 띄워쓰기를 기준으로, 두개의 문자열로 나눠주었습니다.
가장 헷갈리고, 의아한 부분이 if elif elif 로직이었는데
for other in others:
AtoB = gr_records[friend][other][0] # A 가 B 에게 준 것
BtoA = gr_records[friend][other][1] # A 가 B 에게 받은 것
if AtoB > BtoA:
next_month_cnt[friend] += 1
elif AtoB < BtoA:
next_month_cnt[other] += 1
elif AtoB == BtoA :
if present_cnt[friend] > present_cnt[other]:
next_month_cnt[friend] += 1
elif present_cnt[friend] < present_cnt[other]:
next_month_cnt[other] += 1
else:
next_month_cnt[other] += 0
를
if (AtoB != 0 | BtoA != 0) & (AtoB == BtoA):
if AtoB > BtoA:
next_month_cnt[friend] += 1
elif AtoB < BtoA:
next_month_cnt[other] += 1
elif AtoB == BtoA :
if present_cnt[friend] > present_cnt[other]:
next_month_cnt[friend] += 1
elif present_cnt[friend] < present_cnt[other]:
next_month_cnt[other] += 1
else:
next_month_cnt[other] += 0
로 했었는데, if (AtoB != 0 | BtoA != 0) & (AtoB == BtoA): 를 할 필요도 없었는데
이게 무슨 의미가 있을까 ? 라는 의문이 들어서 이해해보려고 했지만
필요 없는 로직이라 그냥 삭제했습니다 ..
아시는 분 계시면, 댓글 달아주세효 ㅠㅠ
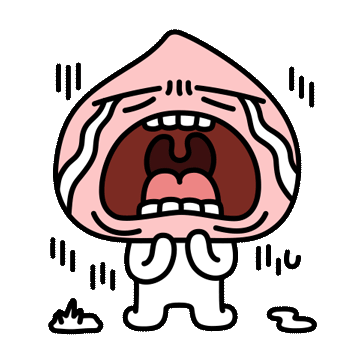
'코딩테스트' 카테고리의 다른 글
[Python][CodingTest] 정수 삼각형 - 동적 계획법(Dynamic Programmging) (0) | 2023.12.28 |
---|---|
[Java] 상품 관리 프로그램 만들기 [기초] (2) | 2023.12.21 |
[Java] 배열+반복문으로 각 학생의 총점, 평균 계산 (0) | 2023.12.20 |
[Python][CodingTest] 달리기 경주 (2) | 2023.12.20 |
[Python] 수열과 구간 쿼리 2, 3 (0) | 2023.12.15 |